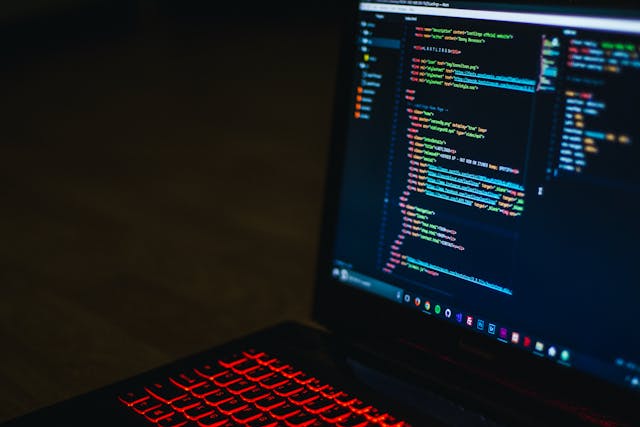
Python is one of the most popular programming languages in the world today, and for good reason. Its simplicity and readability make it an ideal choice for beginners, while its power and versatility attract seasoned developers. Whether you’re looking to start a tech career, automate tedious tasks, or simply pick up a new skill, learning Python is a fantastic place to begin. As a senior developer, I’ve seen firsthand how Python can transform how we approach problems and build solutions. In this guide, I’ll take you through a seven-day journey to mastering the basics of Python.
Day 1: Getting Started
Setting Up Your Environment
The first step in learning Python is setting up your development environment. You can download Python from the official website. Once installed, choose an Integrated Development Environment (IDE) like PyCharm, VSCode, or even Jupyter Notebook. I prefer PyCharm for its robust features and user-friendly interface.
Python Basics
Python’s syntax is straightforward to understand. It uses indentation to define blocks of code, which enhances readability. On your first day, focus on understanding the following basics:
- Variables and Data Types: Learn how to declare variables and work with data types like integers, floats, strings, and booleans.
- Basic Input and Output: Practice using
input()
for getting user input andprint()
for displaying output.
Here’s a simple exercise to get you started:
# This is a simple Python program
name = input("Enter your name: ")
print(f"Hello, {name}!")
Day 2: Control Structures
Conditional Statements
Control structures allow you to dictate the flow of your program. Start with conditional statements:
- If, Elif, Else: Learn how to create conditions using
if
,elif
, andelse
.
age = int(input("Enter your age: "))
if age < 18:
print("You are a minor.")
elif age < 65:
print("You are an adult.")
else:
print("You are a senior.")
Loops
Loops let you execute a block of code multiple times:
- For Loops: Iterate over a sequence (like a list or a range).
- While Loops: Continue looping as long as a condition is true.
# For loop example
for i in range(5):
print(i)
# While loop example
count = 0
while count < 5:
print(count)
count += 1
Day 3: Functions and Modules
Defining and Calling Functions
Functions help you organize and reuse code. On day three, learn how to define and call functions:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Introduction to Modules
Modules are files containing Python code. They allow you to break your program into manageable pieces. Start by importing standard library modules like math
:
import math
print(math.sqrt(16))
Day 4: Data Structures
Lists
Lists are ordered collections of items:
fruits = ["apple", "banana", "cherry"]
fruits.append("date")
print(fruits)
Tuples
Tuples are immutable sequences:
coordinates = (10.0, 20.0)
print(coordinates)
Dictionaries
Dictionaries store key-value pairs:
student = {"name": "John", "age": 21}
print(student["name"])
Sets
Sets are collections of unique items:
numbers = {1, 2, 3, 4, 4}
print(numbers) # Output: {1, 2, 3, 4}
Day 5: File Handling and Exceptions
Reading and Writing Files
Learn how to handle files to store and retrieve data:
# Writing to a file
with open("example.txt", "w") as file:
file.write("Hello, world!")
# Reading from a file
with open("example.txt", "r") as file:
content = file.read()
print(content)
Exception Handling
Errors are inevitable, but Python provides ways to handle them gracefully:
try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
finally:
print("This runs no matter what.")
Day 6: Object-Oriented Programming (OOP)
Introduction to OOP Concepts
OOP is a powerful paradigm that allows you to model real-world entities:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
return "Woof!"
my_dog = Dog("Buddy", 3)
print(my_dog.bark())
Inheritance and Polymorphism
Inheritance allows you to create new classes based on existing ones:
class Animal:
def speak(self):
pass
class Cat(Animal):
def speak(self):
return "Meow"
my_cat = Cat()
print(my_cat.speak())
Day 7: Advanced Topics and Final Project
Introduction to Libraries and Frameworks
Python’s ecosystem is rich with libraries and frameworks that extend its capabilities:
- NumPy: For numerical operations.
- Pandas: For data analysis.
- Flask: For web development.
Explore these libraries to understand their basic usage.
Working on a Final Project
Choose a project that incorporates the concepts you’ve learned. Here’s a simple project idea:
Project: To-Do List Application
- Project Planning: Define the features (adding tasks, marking tasks as done, viewing tasks).
- Implementation: Write the code using functions, file handling, and possibly a simple GUI with Tkinter.
- Testing and Debugging: Ensure your application works as expected.
Review and Next Steps
Recap what you’ve learned and explore additional resources to deepen your knowledge:
- Books: “Automate the Boring Stuff with Python” by Al Sweigart.
- Online Courses: Coursera, Udacity, and edX offer excellent Python courses.
- Community Resources: Join Python communities like Stack Overflow and Reddit to ask questions and share your knowledge.
Conclusion
Learning Python in just seven days is an ambitious goal, but it’s entirely achievable with dedication and the right approach. Python’s simplicity and vast community support make it an ideal language for beginners. As you continue your journey, remember that practice is key. Keep experimenting with new projects, and don’t hesitate to seek help from the Python community. Happy coding!
References
For further reading and insights, consider exploring:
- Python documentation at python.org
- Online tutorials and coding challenges at HackerRank
- Community forums like Stack Overflow
By staying informed and engaged, you’ll be well on mastering Python and leveraging it to build incredible applications.